1.7. Iteration#
1.7.1. The while
statement#
In Python, the while
statement is used to create a loop that executes a block of code repeatedly as long as a certain condition remains true.
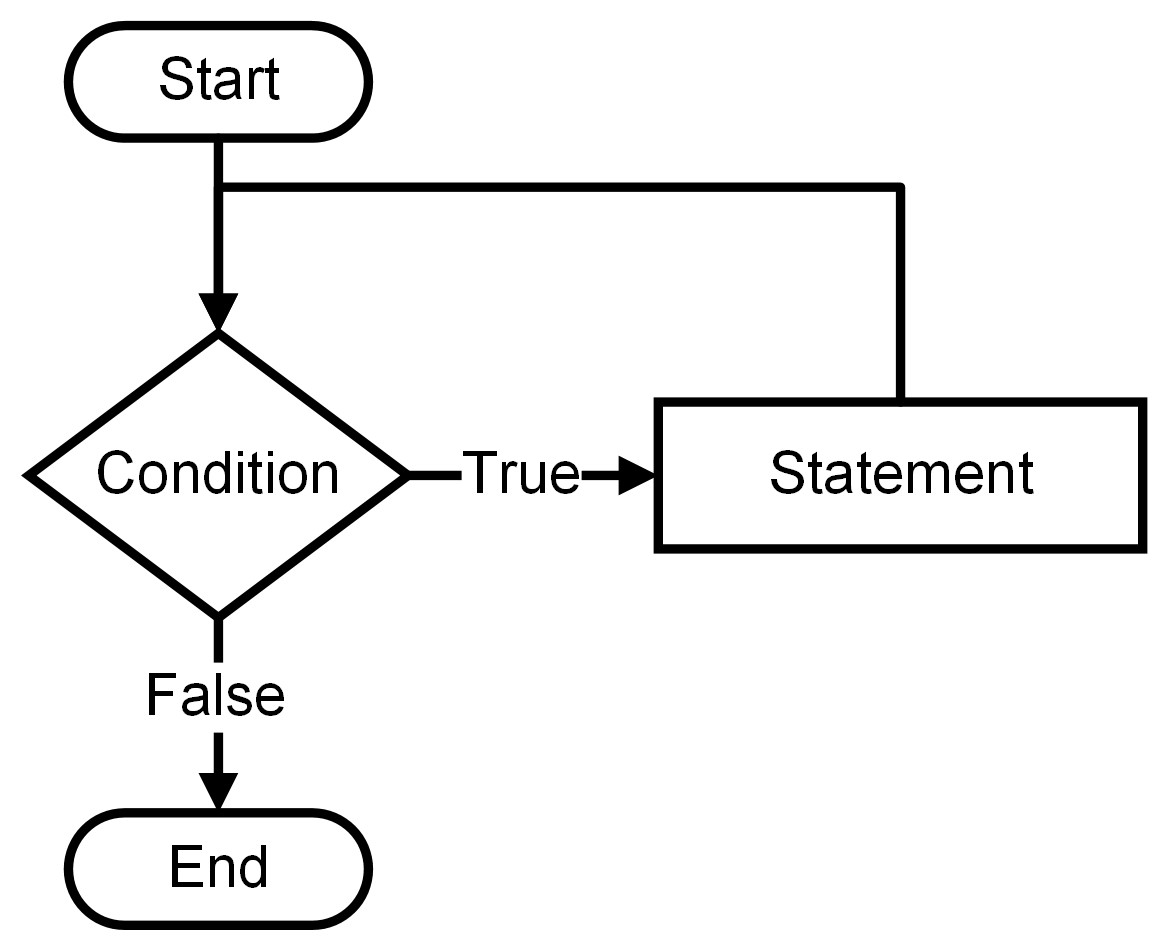
Fig. 1.8 Flowchart: The Structure of a ‘While’ Loop in Python.#
The general syntax of the while
loop is as follows:
while condition:
# Code block to be executed while the condition is True
# ...
The loop will keep executing the code block as long as the condition
remains true. Once the condition
becomes false, the loop will terminate, and the program will continue with the next line of code after the while
loop.
A simple example of using a while
loop to count from 1 to 5:
count = 1
while count <= 5:
print(count)
count += 1
1
2
3
4
5
Fig. 1.9 visualizes the iterative process of a while
loop, structured into five rows to clearly represent the flow of execution.
Start Node: The flowchart begins at the “Start” node, indicating the entry point of the loop.
Initialize Node: The “Initialize” node represents the initialization of the variable
count
to 1.Condition Node: The diamond-shaped “Condition” node checks whether
count <= 5
:If true, the flow proceeds to the “Print” and “Increment” nodes.
Print and Increment Nodes: These nodes are aligned in the same row:
Print Node: Represents the action of printing the current value of
count
.Increment Node: Increases the value of
count
by 1 and loops back to the “Condition” node to re-evaluate the condition.
End Node: When the condition
count <= 5
is false, the flowchart concludes at the “End” node, marking the completion of the loop.
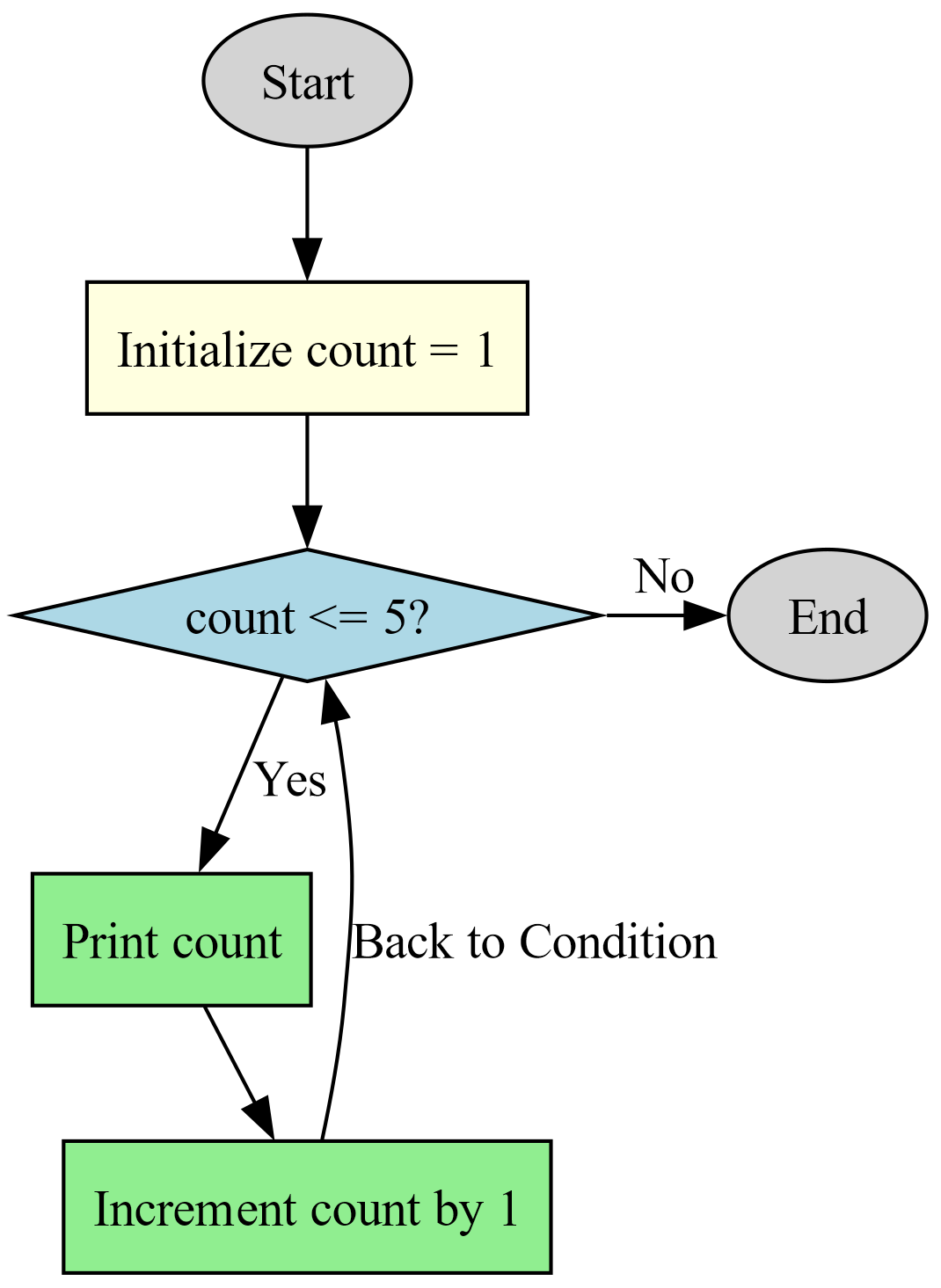
Fig. 1.9 Flowchart of the while
loop from the above example.#
It’s important to ensure that the condition in the while
loop eventually becomes false; otherwise, the loop will result in an infinite loop, causing the program to run indefinitely. You should include logic in the loop to modify the variables involved in the condition so that the condition becomes false at some point [Downey, 2015, Python Software Foundation, 2024].
An example of an infinite loop:
# Infinite loop (remove this code after running, as it will keep running indefinitely)
while True:
print("This is an infinite loop!")
In this example, the loop condition True
is always true, so the loop will never terminate, and “This is an infinite loop!” will be printed indefinitely. To stop the program from running, you can manually interrupt the execution (e.g., press Ctrl + C in the terminal or stop the program execution in the IDE).
1.7.2. break
#
In Python, the break
statement is used to terminate the execution of a loop prematurely. When the break
statement is encountered within a loop, the loop is immediately exited, and the program continues with the first statement after the loop [Downey, 2015, Python Software Foundation, 2024].
The break
statement is often used in combination with conditional statements (such as if
) to exit the loop based on a specific condition.
Here’s an example of using break
to stop a loop when a certain condition is met:
# Using break to stop the loop when the value becomes 5
count = 1
while count <= 10:
print(count)
if count == 5:
break
count += 1
1
2
3
4
5
Fig. 1.10 visualizes the iterative process of a while
loop with a break
statement, structured into five rows to clearly represent the flow of execution.
Start Node: The flowchart begins at the “Start” node, indicating the entry point of the loop.
Initialize Node: The “Initialize” node represents the initialization of the variable
count
to 1.Condition Node: The diamond-shaped “Condition” node checks whether
count <= 10
:If true, the flow proceeds to the “Print” node.
Print, Break Condition, and Increment Nodes: These nodes are aligned in the same row:
Print Node: Represents the action of printing the current value of
count
.Break Condition Node: Checks if
count == 5
.If true, the flow goes to the “Break” node, which exits the loop.
If false, the flow proceeds to the “Increment” node.
Increment Node: Increases the value of
count
by 1 and loops back to the “Condition” node to re-evaluate the condition.
End Node: When the loop is exited (either by the
break
statement or whencount > 10
), the flowchart concludes at the “End” node, marking the completion of the loop.
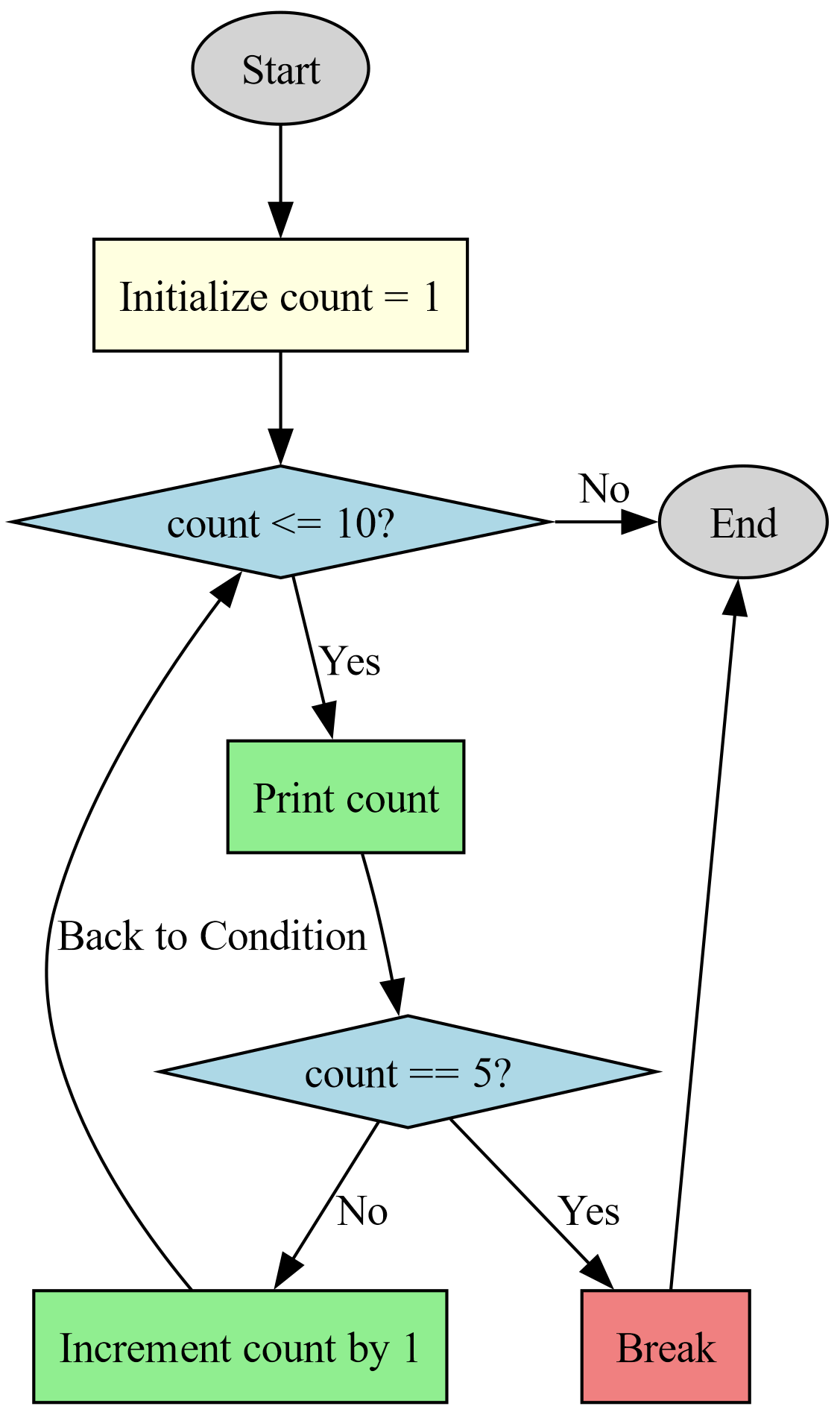
Fig. 1.10 Flowchart of the while
loop with a break
statement from the above example.#
numbers = [1, 2, 3, 4, 5]
for num in numbers:
print(num)
1
2
3
4
5
In this example, the for
loop iterates over each element in the list numbers
. For each iteration, the variable num
takes the value of the current element, and we print it on a separate line.
We can also use the for
loop to iterate over a string:
text = "Hello, Calgary!"
for char in text:
print(char)
H
e
l
l
o
,
C
a
l
g
a
r
y
!
Fig. 1.11 visualizes the process of a for
loop iterating over each character in a string, structured to clearly represent the flow of execution.
Start Node: The flowchart begins at the “Start” node, indicating the entry point of the loop.
Initialize Node: The “Initialize” node represents the initialization of the variable
text
with the string “Hello, Calgary!”.For Loop Node: The diamond-shaped “ForLoop” node iterates over each character in the string
text
:For each character, the flow proceeds to the “Print” node.
Print Node: Represents the action of printing the current character
char
.Loop Back: After printing, the flow returns to the “ForLoop” node to process the next character.
End Node: When all characters have been processed, the flowchart concludes at the “End” node, marking the completion of the loop.
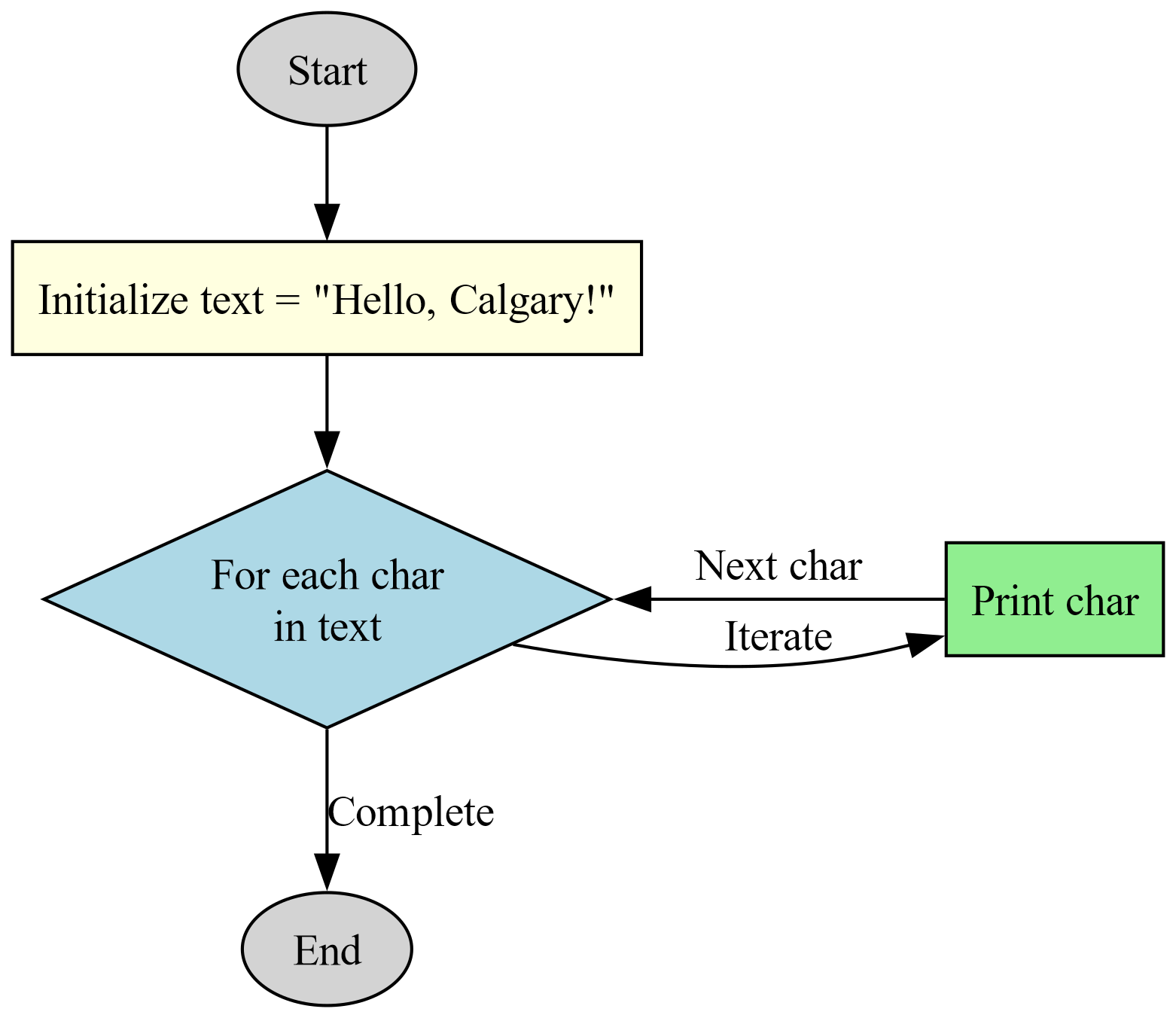
Fig. 1.11 Flowchart of the for
loop iterating over each character in the string “Hello, Calgary!”.#
The for
loop is not limited to lists and strings; you can use it with any iterable object, including tuples, dictionaries, and even custom objects that support iteration.
In the case of dictionaries, you can use the for
loop to iterate over keys, values, or items (key-value pairs):
student_scores = {"Alice": 85, "Bob": 92, "Charlie": 78}
for name,score in student_scores.items():
print(f"{name}: {score}")
Alice: 85
Bob: 92
Charlie: 78
Fig. 1.12 visualizes the process of a for
loop iterating over each key-value pair in a dictionary, with increased spacing between edges to prevent label overlap.
Start Node: The flowchart begins at the “Start” node, indicating the entry point of the loop.
Initialize Node: The “Initialize” node represents the initialization of the dictionary
student_scores
with the given key-value pairs.For Loop Node: The diamond-shaped “ForLoop” node iterates over each key-value pair in the dictionary
student_scores
:For each pair, the flow proceeds to the “Print” node.
Print Node: Represents the action of printing the current
name
andscore
in the format"{name}: {score}"
.Loop Back: After printing, the flow returns to the “ForLoop” node to process the next key-value pair.
End Node: When all key-value pairs have been processed, the flowchart concludes at the “End” node, marking the completion of the loop.
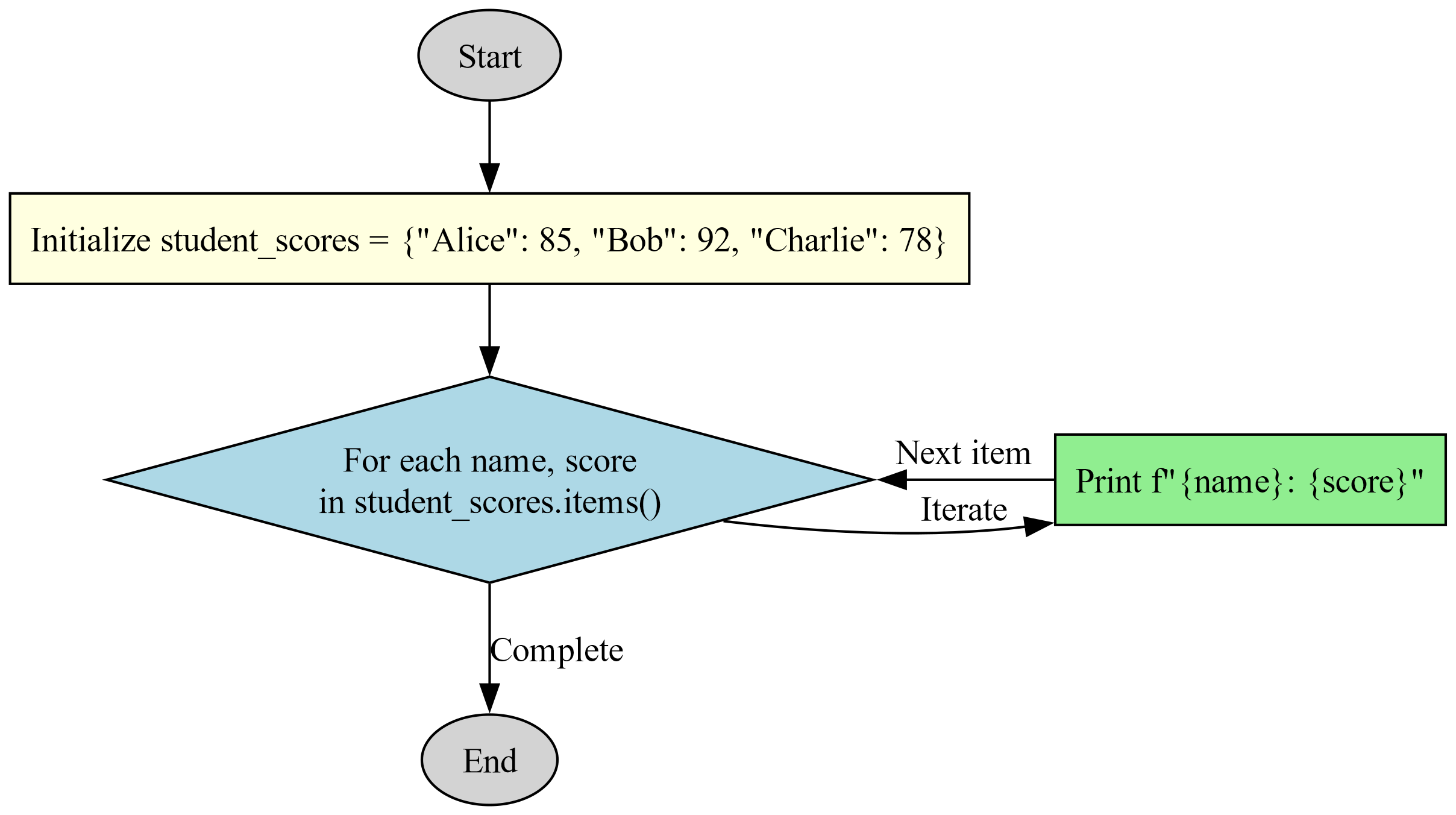
Fig. 1.12 Flowchart of the for
loop iterating over each key-value pair in the student_scores
dictionary.#
The for
loop is a powerful and versatile tool in Python for processing sequences and collections of data. It simplifies the process of iterating over elements and is commonly used in various programming tasks.
Summary:
Loop Type |
Description |
---|---|
|
Executes a block of code a specified number of times, iterating over a sequence (such as a list, tuple, or range) or any iterable object. |
|
Repeatedly executes a block of code as long as a given condition is true. The loop continues until the condition becomes false. |
Note
Discussion on dictionaries will be covered in Section 2.4.