1.6. Conditionals#
Conditionals are the foundation of decision-making in programming. Through conditional statements like “if,” “else,” and “elif,” programmers can direct the program’s execution based on different conditions.
1.6.1. Conditional execution#
In Python, conditional execution allows you to execute specific blocks of code based on certain conditions. This is typically achieved using the if
, elif
(optional), and else
(optional) statements. The general syntax for conditional execution in Python is as follows [Downey, 2015, Python Software Foundation, 2024]:
if condition:
# Code block to be executed if the condition is True
elif condition2: # Optional (elif stands for "else if")
# Code block to be executed if condition2 is True (only if the preceding 'if' is False)
else: # Optional
# Code block to be executed if none of the above conditions are True
Fig. 1.1 illustrates the decision-making process of an if-elif-else
structure, showing how different conditions lead to specific code blocks being executed.
Start Node: The flowchart begins at the “Start” node, represented as an ellipse, indicating the entry point of the decision-making process.
Decision Node (Condition 1): The first diamond-shaped node represents the check for “condition 1”:
If “condition 1” is true, the flow proceeds to the “TrueBlock” node, executing the corresponding code block.
Elif Decision Node (Condition 2): If “condition 1” is false, the flow moves to the second decision node for “condition 2”:
If “condition 2” is true, the flow proceeds to the “ElifBlock” node, executing the corresponding code block.
Else Block: If neither “condition 1” nor “condition 2” is true, the flow moves to the “ElseBlock” node, executing the code block associated with the
else
clause.End Node: The flowchart concludes at the “End” node, marking the completion of the decision-making process after executing the appropriate code block.
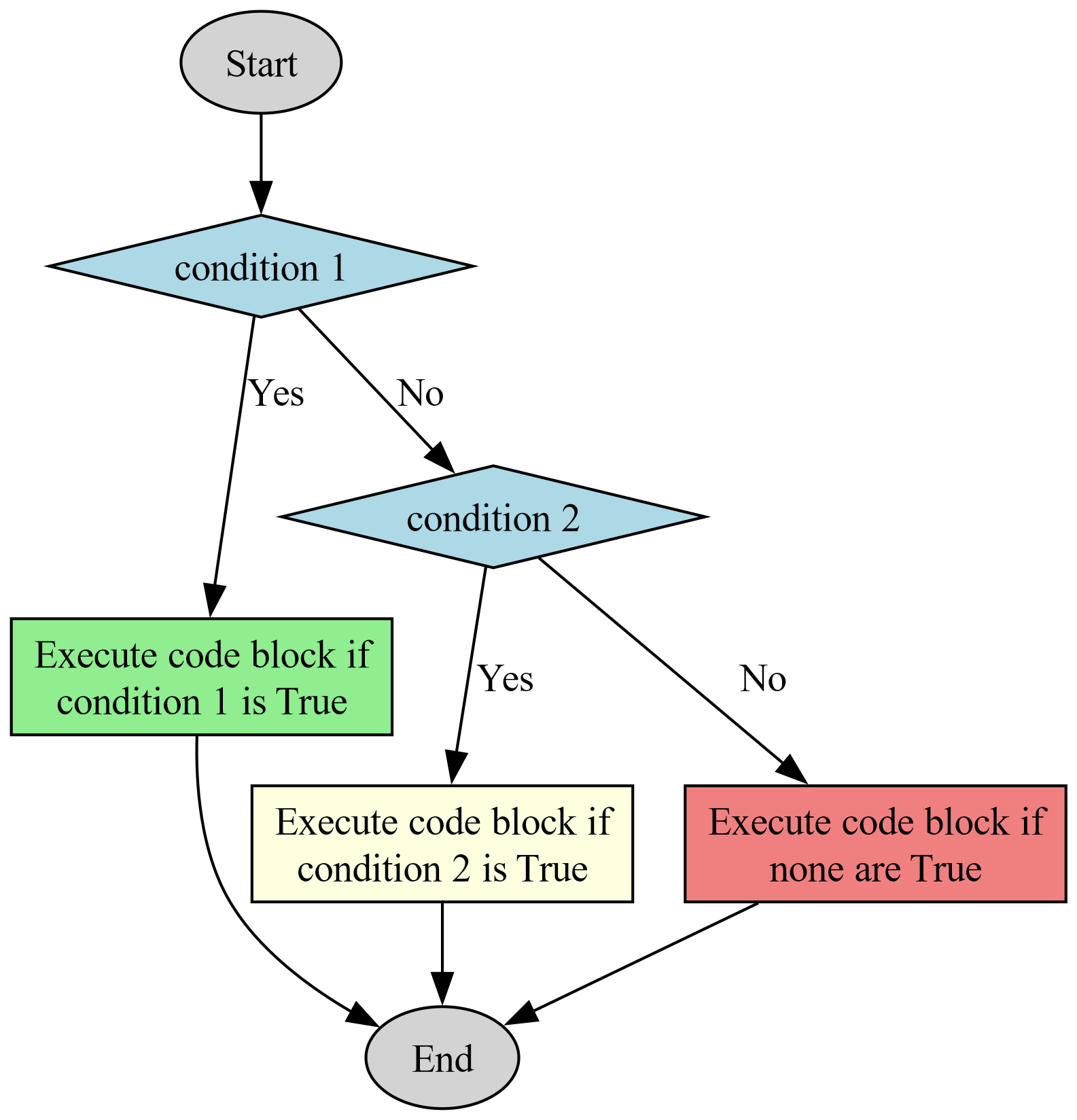
Fig. 1.1 Flowchart of an if-elif-else
Structure.#
Let’s look at some examples to better understand conditional execution:
1.6.1.1. Using if
statement only#
x = 10
if x > 5:
print("x is greater than 5.")
x is greater than 5.
In this example, if the value of x
is greater than 5, the code block under the if
statement will be executed, and “x is greater than 5.” will be printed.
Fig. 1.2 visualizes the logic of a Python program that checks if a variable x
is greater than 5 and prints a message if the condition is true.
Start Node:
The flowchart begins at the “Start” node, represented as an ellipse, indicating the entry point of the program.
Decision Node:
The “Decision” node, shaped as a diamond, represents the conditional check
x > 5?
.This node evaluates whether the variable
x
is greater than 5.
Print Node:
The “Print” node, depicted as a rectangle, is positioned to the left of the “Decision” node.
If the condition
x > 5
is true, the program executes the actionprint("x is greater than 5.")
.An edge labeled “Yes” connects the “Decision” node to the “Print” node, indicating the path taken when the condition is satisfied.
End Node:
The “End” node, another ellipse, marks the conclusion of the program.
If the condition
x > 5
is false, the flowchart directly connects the “Decision” node to the “End” node via an edge labeled “No.”After executing the print statement, the flow continues to the “End” node, indicating the program’s completion.
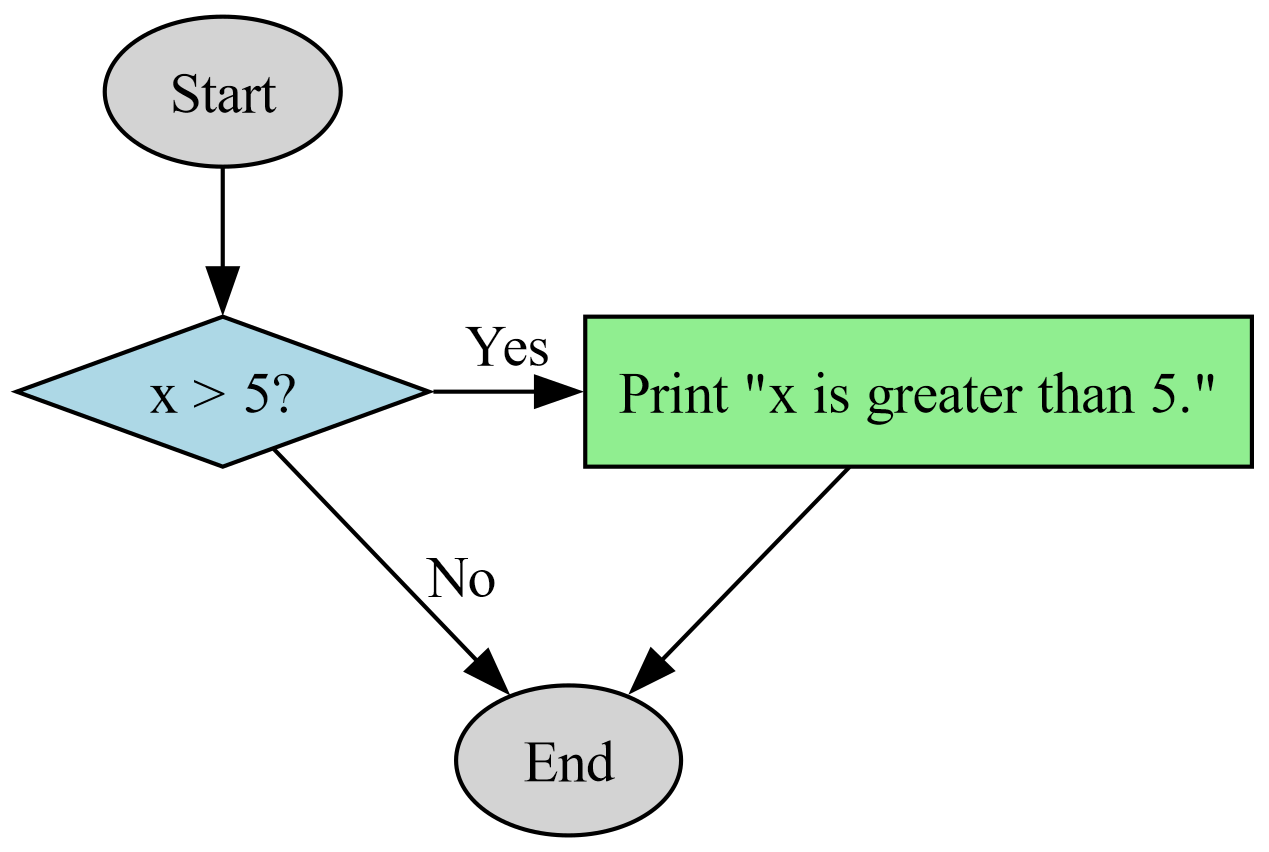
Fig. 1.2 Flowchart of a Simple Conditional Statement.#
1.6.1.2. Using if
and else
statements#
x = 3
if x > 5:
print("x is greater than 5.")
else:
print("x is not greater than 5.")
x is not greater than 5.
In this example, if the value of x
is greater than 5, the code block under the if
statement will be executed. Otherwise, the code block under the else
statement will be executed.
Fig. 1.3 illustrates the logic of a Python program that checks if a variable x
is greater than 5 and executes different print statements based on the condition’s outcome.
Start Node:
The flowchart starts at the “Start” node, represented as an ellipse, indicating the entry point of the program.
Decision Node:
The “Decision” node, shaped as a diamond, represents the conditional check
x > 5?
.This node evaluates whether the variable
x
is greater than 5.
Print Nodes:
Two “Print” nodes are used to represent the actions taken based on the decision:
PrintYes: If the condition
x > 5
is true, the program executes the actionprint("x is greater than 5.")
. This node is positioned to the left of the “Decision” node.PrintNo: If the condition is false, the program executes the action
print("x is not greater than 5.")
. This node is positioned to the right of the “Decision” node.
Edges labeled “Yes” and “No” connect the “Decision” node to the respective “Print” nodes, indicating the paths taken based on the condition’s evaluation.
End Node:
The “End” node, another ellipse, marks the conclusion of the program.
After executing either print statement, the flow continues to the “End” node, indicating the program’s completion.
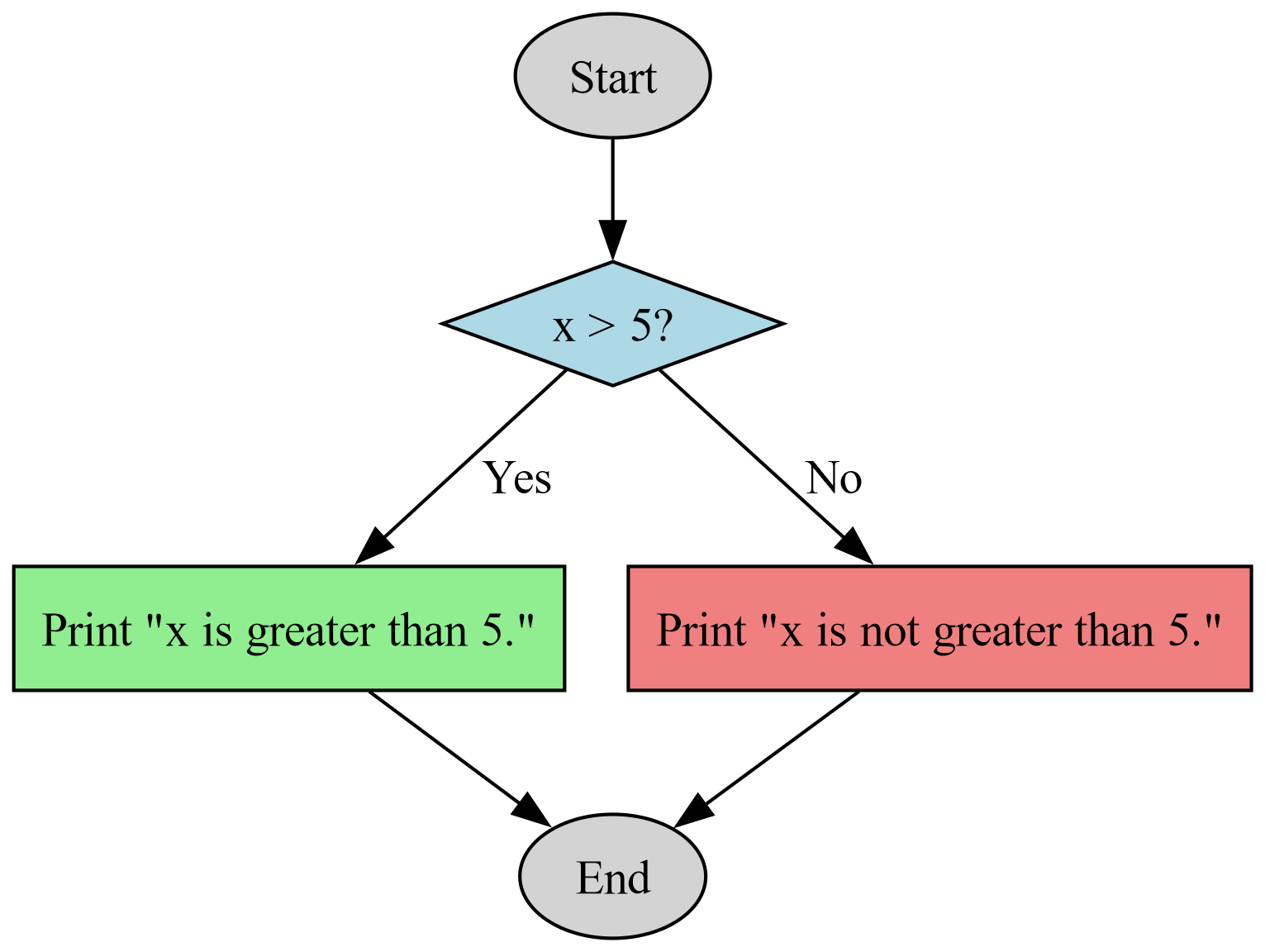
Fig. 1.3 Flowchart of a Conditional Statement with an else
Clause.#
1.6.1.3. Using if
, elif
, and else
statements#
Example:
x = 5
if x > 5:
print("x is greater than 5.")
elif x == 5:
print("x is equal to 5.")
else:
print("x is less than 5.")
x is equal to 5.
In this example, if the value of x
is greater than 5, the first if
block will be executed. If x
is equal to 5, the elif
block will be executed. If both conditions are False, the else
block will be executed.
Fig. 1.4 visualizes the logic of a Python program that checks the value of a variable x
and executes different print statements based on whether x
is greater than, equal to, or less than 5.
Start Node:
The flowchart begins at the “Start” node, represented as an ellipse, indicating the entry point of the program.
Decision Node:
The “Decision” node, shaped as a diamond, represents the conditional check
x > 5?
.This node evaluates whether the variable
x
is greater than 5.
Conditional Branches:
Print Greater: If the condition
x > 5
is true, the program executes the actionprint("x is greater than 5.")
. This node is positioned on the third row to the left.Check Equal: If
x > 5
is false, the program checksx == 5?
in another decision node. This node is positioned in the center of the third row.Print Equal: If
x == 5
is true, the program executesprint("x is equal to 5.")
.Print Less: If both conditions are false (
x > 5
andx == 5
), the program executesprint("x is less than 5.")
. This node is positioned on the third row to the right.
End Node:
The “End” node, another ellipse, marks the conclusion of the program.
After executing any of the print statements, the flowchart directs to the “End” node, indicating the program’s completion.
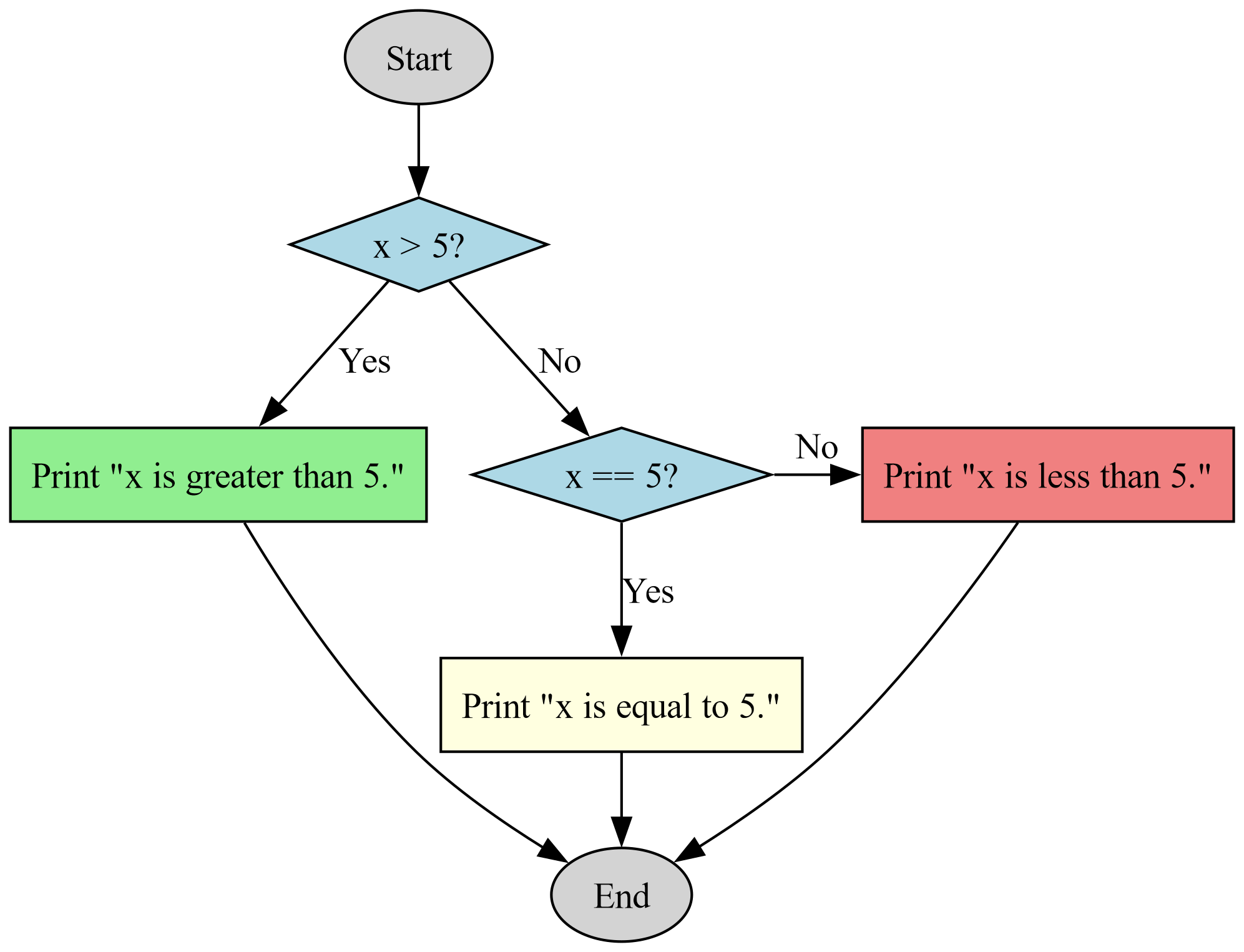
Fig. 1.4 Flowchart of a Conditional Statement with if
, elif
, and else
Clauses.#
Conditional execution is fundamental for making decisions in programming. It allows your code to respond to different situations and execute appropriate actions accordingly. You can have nested if-elif-else structures to handle more complex decision-making scenarios.
Example: Consider an illustrative case of nested conditionals that ascertain a student’s grade based on their score. This process is demonstrated using the following link: https://conted.ucalgary.ca/info/grades.jsp
def get_letter_grade(score):
if score >= 95:
return "A+ - Outstanding"
elif score >= 90:
return "A - Excellent\nSuperior performance, showing comprehensive understanding of subject matter."
elif score >= 85:
return "A- - Approaching Excellent"
elif score >= 80:
return "B+ - Exceeding Good"
elif score >= 75:
return "B - Good\nClearly above average performance with knowledge of subject matter generally complete."
elif score >= 70:
return "B- - Approaching Good"
elif score >= 67:
return "C+ - Exceeding Satisfactory"
elif score >= 64:
return "C - Satisfactory (minimal pass)\nBasic understanding of subject matter. Minimum required in all courses to meet certificate program requirements."
elif score >= 60:
return "C- - Approaching Satisfactory\nReceipt of a C- or less is not sufficient for certificate program requirements."
elif score >= 55:
return "D+ - Marginal Performance"
elif score >= 50:
return "D - Minimal Performance"
else:
return "F - Fail"
score = 85
print(get_letter_grade(score))
A- - Approaching Excellent
This code essentially acts as a grading scale and provides descriptive information about the grade based on the input score. Here’s a brief explanation of the code:
The
get_letter_grade
function takes a single parameter,score
, which is expected to be a numerical value representing a student’s score.The function uses a series of conditional statements (if, elif, else) to determine the appropriate letter grade based on the value of the
score
.Each conditional branch specifies a certain score range along with a corresponding letter grade and a brief description of that grade. For example, if the
score
is 95 or above, the function returns “A+ - Outstanding”. If thescore
is between 90 and 94, it returns “A - Excellent” along with a more detailed description of excellent performance.The code example at the bottom sets the
score
variable to 85 and then calls theget_letter_grade
function with this score. The result is printed using theprint
function.In this specific example, with a
score
of 85, the function returns “A- - Approaching Excellent”.
Fig. 1.5 visualizes how the get_letter_grade
function assigns letter grades based on a numerical score, detailing the decision-making process for various score thresholds.
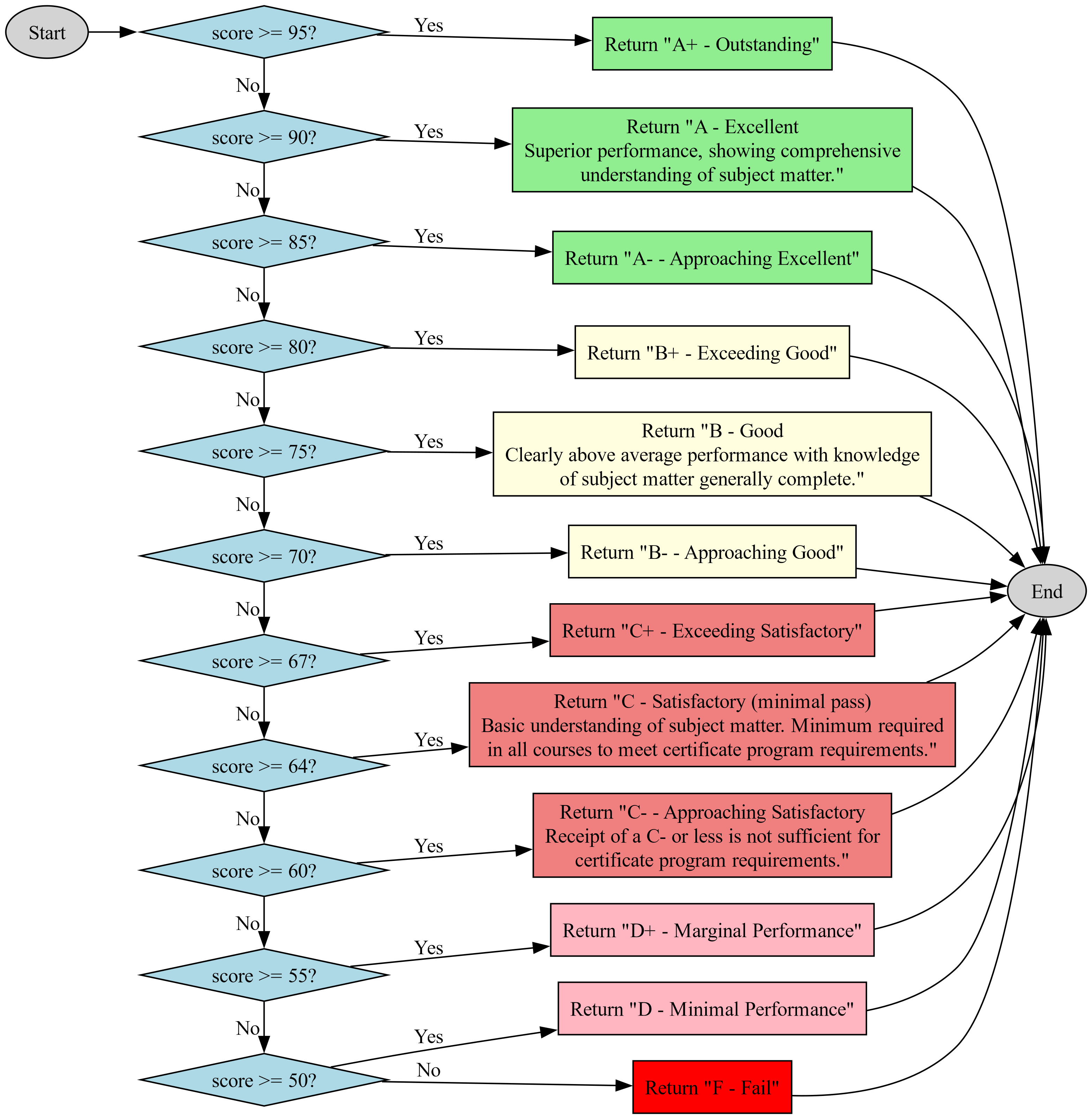
Fig. 1.5 Flowchart of the get_letter_grade
Function.#
1.6.2. Nested conditionals#
In Python, nested conditionals refer to the practice of placing conditional statements (if, elif, else) inside other conditional statements. This allows you to create more complex decision-making structures and hierarchically handle multiple conditions. The syntax for nested conditionals is as follows:
if condition1:
# Code block to be executed if condition1 is True
if nested_condition1:
# Code block to be executed if both condition1 and nested_condition1 are True
elif nested_condition2:
# Code block to be executed if condition1 is True and nested_condition2 is True
else:
# Code block to be executed if condition1 is True but none of the nested conditions are True
elif condition2:
# Code block to be executed if condition1 is False and condition2 is True
if nested_condition3:
# Code block to be executed if condition2 is True and nested_condition3 is True
else:
# Code block to be executed if condition2 is True but nested_condition3 is False
else:
# Code block to be executed if both condition1 and condition2 are False
Fig. 1.6 visualizes the logic of a nested conditional structure, showing how different conditions and nested conditions lead to specific code blocks being executed.
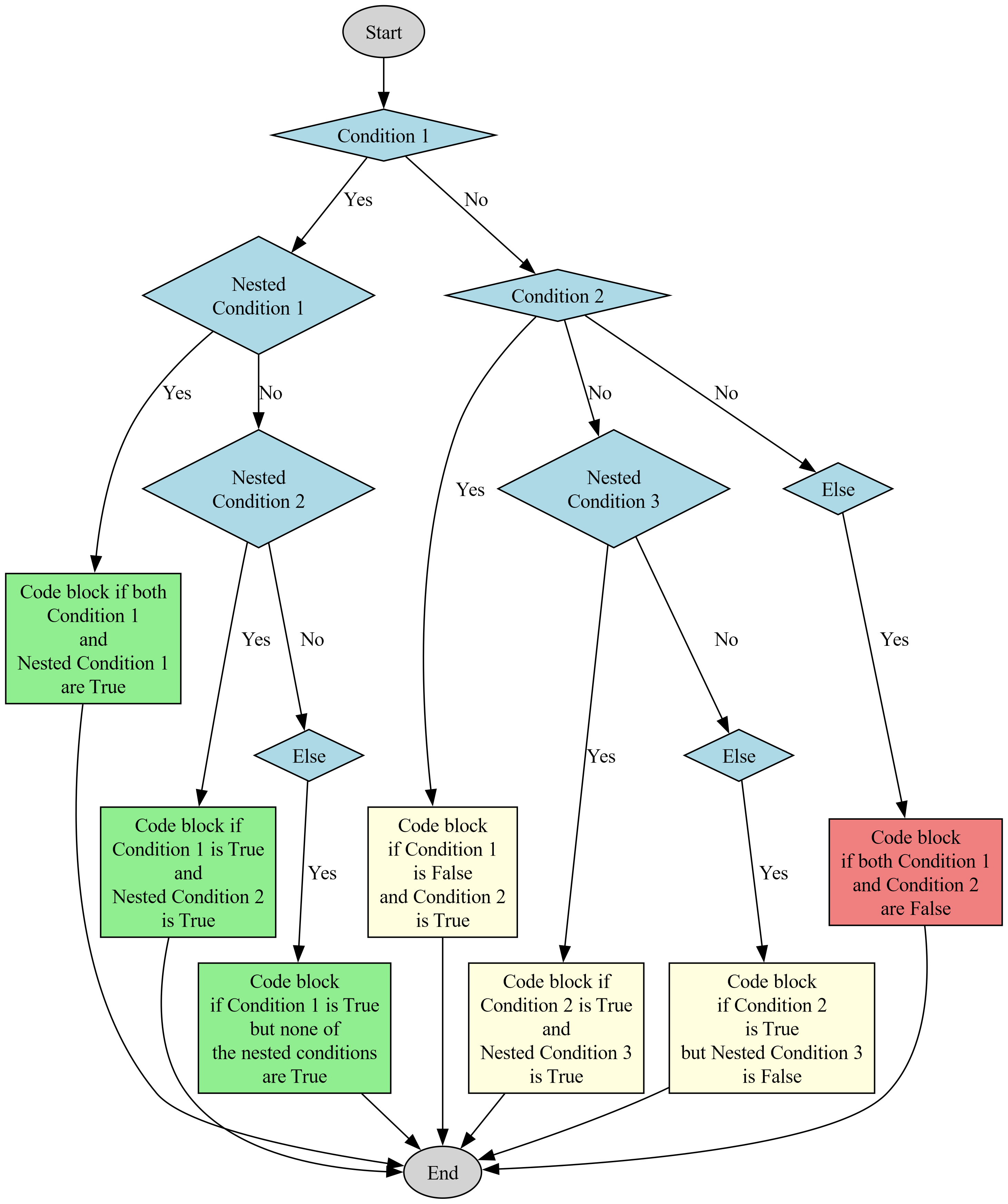
Fig. 1.6 Flowchart of nested conditional statements#
Example: Here’s an example of nested conditional statements as per your provided structure:
# Assign values to variables
x = 10
y = 5
# Check if x is greater than y
if x > y:
print("x is greater than y") # Print this if x is greater than y
# Check if x is greater than 15
if x > 15:
print("x is also greater than 15") # Print this if x is greater than 15
# Check if x is greater than 12 but not greater than 15
elif x > 12:
print("x is greater than 12 but not greater than 15") # Print this if x is between 12 and 15
else:
print("x is greater than y, but none of the nested conditions matched") # Print this if none of the above conditions in this block match
# Check if x is less than y
elif x < y:
print("x is less than y") # Print this if x is less than y
# Check if y is less than 8
if y < 8:
print("y is less than 8") # Print this if y is less than 8
else:
print("y is not less than 8") # Print this if y is 8 or greater
# If neither of the above conditions are met
else:
print("x and y are equal") # Print this if x and y have the same value
x is greater than y
x is greater than y, but none of the nested conditions matched
In this example:
We first compare
x
andy
using the outermostif
,elif
, andelse
structure.If
x
is greater thany
, we enter the nestedif
,elif
, andelse
structure inside that block.Depending on the value of
x
, we print different messages within the nested structure.If
x
is less thany
, we enter the nested structure under theelif
block for the case wherex
is less thany
.Finally, if neither of the outer conditions is met, we execute the code in the
else
block.
This demonstrates the nesting of conditional statements, where the behavior of the program depends on multiple levels of conditions and nested conditions.
Fig. 1.7 illustrates the logic of a Python program that compares two variables, x
and y
, and executes print statements based on various conditions.
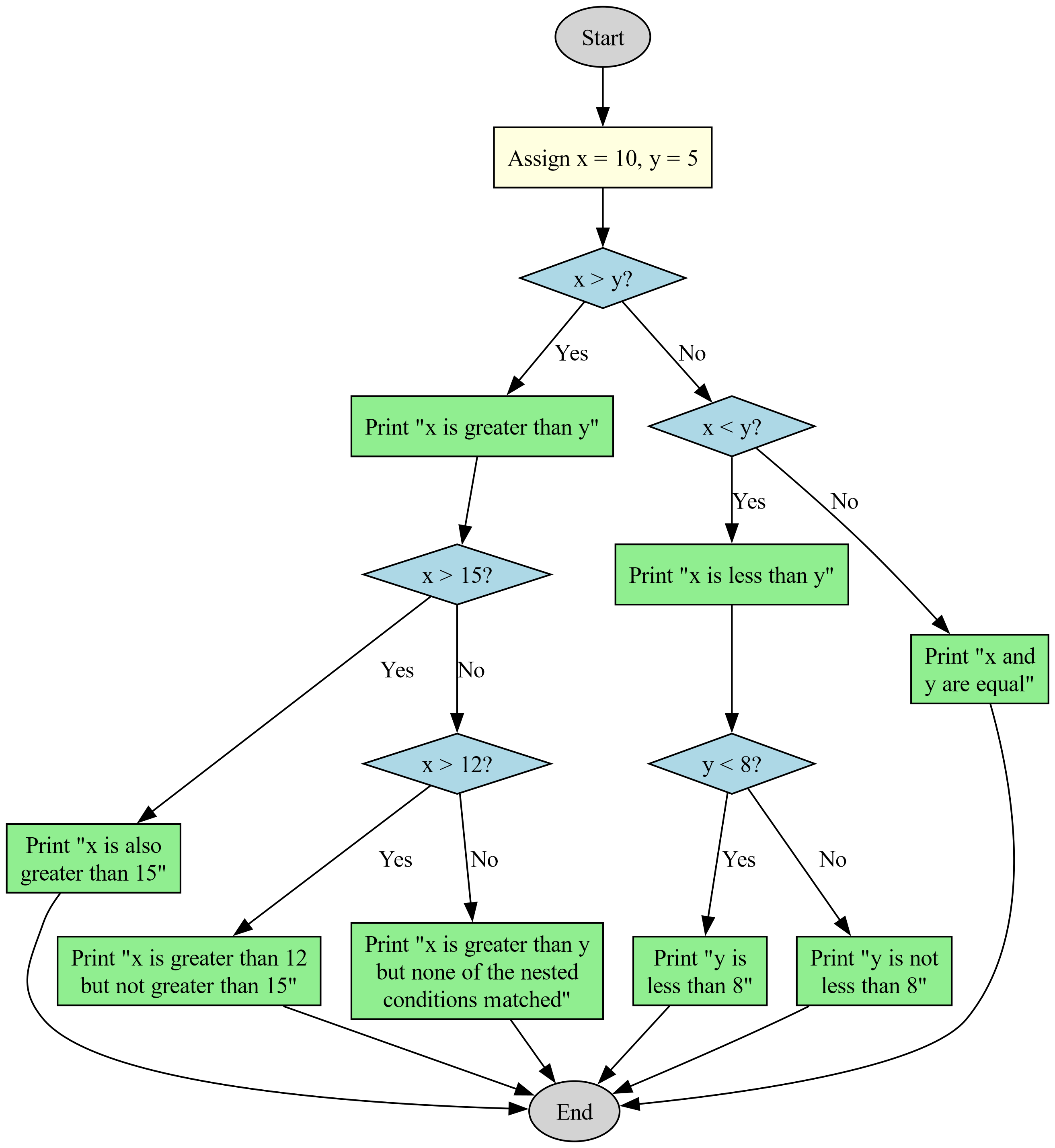
Fig. 1.7 Flowchart of the example nested conditional statement#