3.3. Python Dictionaries: Key-Value Pairs in Python#
3.3.1. A dictionary is a Mapping#
In Python, a dictionary is a data structure that represents a collection of key-value pairs. It is also known as an associative array, hash map, or hash table in other programming languages. Dictionaries are mutable, which means you can modify their contents after they are created. They are particularly useful when you need to store and retrieve data based on a unique identifier (the key) [Downey, 2015, Python Software Foundation, 2023].
In a dictionary, each key is unique and maps to a specific value. The keys must be immutable data types (e.g., strings, numbers, tuples), while the values can be of any data type (e.g., strings, numbers, lists, other dictionaries).
Example: Here’s how you can create a dictionary in Python:
# Method 1: Using curly braces and colons to define key-value pairs
person = {"Name": "John",
"Age": 35,
"City": "Calgary"
}
# Method 2: Using the dict() constructor
person = dict(name="John", age=35, city=" Calgary ")
# Method 3: Using a list of tuples and the dict() constructor
person_info = [("Name", "John"), ("Age", 35), ("City", "Calgary")]
person = dict(person_info)
print(person)
{'Name': 'John', 'Age': 35, 'City': 'Calgary'}
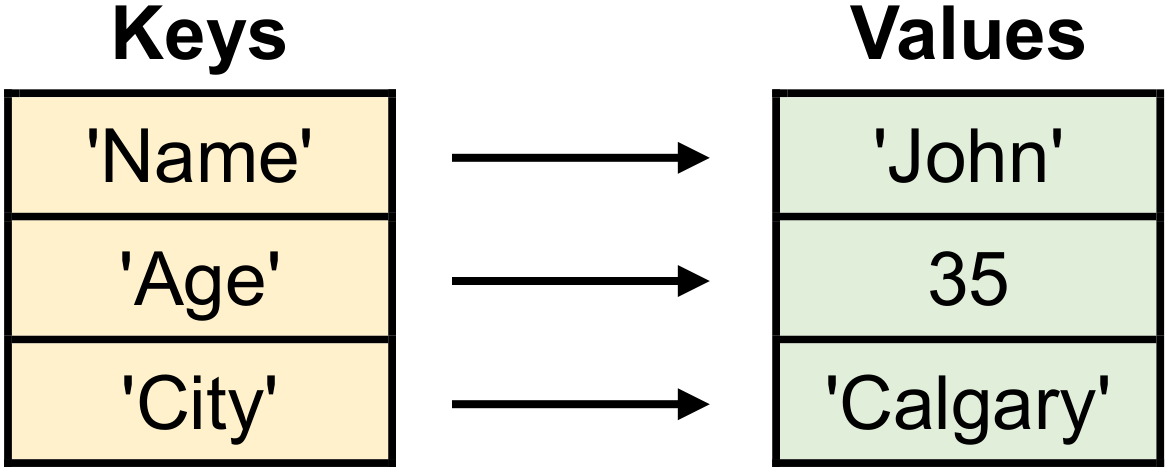
Fig. 3.7 A visual representation of the defined dictionary .#
You can access values in the dictionary by using their corresponding keys:
# Create a dictionary representing a person's information
person = {"Name": "John",
"Age": 35,
"City": "Calgary"}
# Access and print specific values from the dictionary
print(f'Name = {person["Name"]}')
print(f'Age = {person["Age"]}')
print(f'City = {person["City"]}')
Name = John
Age = 35
City = Calgary
You can also modify, add, or remove key-value pairs in the dictionary:
# Modify the value associated with the "Age" key
person["Age"] = 31
# Add a new key-value pair to the dictionary
person["Occupation"] = "Engineer"
# Remove a key-value pair from the dictionary using the "City" key
del person["City"]
# Print the updated dictionary
print(person)
{'Name': 'John', 'Age': 31, 'Occupation': 'Engineer'}
3.3.2. Iterating Over Dictionaries#
In Python, you can loop through dictionaries using various methods to access and work with their key-value pairs. Here are some common ways to loop through dictionaries [Downey, 2015, Python Software Foundation, 2023]:
3.3.2.1. Looping through keys using for
loop#
Example:
# Create a dictionary with key-value pairs representing a person's information
my_dict = {"Name": "John", "Age": 35, "City": "Calgary", "Occupation": "Engineer"}
# Iterate through the keys in the dictionary and print each key-value pair
for key in my_dict:
print(key, ':', my_dict[key])
Name : John
Age : 35
City : Calgary
Occupation : Engineer
Here’s what each part of the code does:
my_dict = {"Name": "John", "Age": 35, "City": "Calgary", "Occupation": "Engineer"}
: This line initializes a Python dictionary namedmy_dict
with key-value pairs. Each key represents a piece of information about a person, such as “Name,” “Age,” “City,” and “Occupation.” The associated values are specific to this individual.for key in my_dict:
: This line initiates afor
loop that iterates through the keys of the dictionarymy_dict
. During each iteration, it assigns the current key to the variablekey
.key
: This variable holds the current key from the dictionary during each iteration.
3.3.2.2. Looping through keys using keys()
method#
Example:
# Iterate through the keys of the dictionary using the keys() method and print each key-value pair
for key in my_dict.keys():
print(key, ':', my_dict[key])
Name : John
Age : 35
City : Calgary
Occupation : Engineer
for key in my_dict.keys():
: This line initiates a for
loop that iterates through the keys of the dictionary my_dict
. In each iteration, it assigns the current key to the variable key
.
key
: This variable holds the current key from the dictionary during each iteration.
3.3.2.3. Looping through values using values()
method#
Example:
# Iterate through the values of the dictionary using the values() method and print each value
for value in my_dict.values():
print(value)
John
35
Calgary
Engineer
for value in my_dict.values():
: This line initiates a for
loop that iterates through the values of the dictionary my_dict
. In each iteration, it assigns the current value to the variable value
.
value
: This variable holds the current value from the dictionary during each iteration.
3.3.2.4. Looping through key-value pairs using items()
method#
Example:
# Iterate through key-value pairs in the dictionary using the items() method and print each key and value
for key, value in my_dict.items():
print(key, ':', value)
Name : John
Age : 35
City : Calgary
Occupation : Engineer
for key, value in my_dict.items():
: This line initiates a for
loop that iterates through the items of the dictionary my_dict
. In each iteration, it unpacks the key-value pair into two variables, key
and value
.
key
: This variable holds the current key in the dictionary during each iteration.value
: This variable holds the corresponding value associated with the current key.
3.3.2.5. Using a list comprehension to manipulate the dictionary#
# Create a dictionary with key-value pairs representing a person's information
my_dict = {"Name": "John", "Age": 35, "City": "Calgary", "Occupation": "Engineer"}
# For example, let's create a new dictionary where age is increased by 1 for each person
# Iterate through key-value pairs in the original dictionary, updating the "Age" key if found
new_dict = {key: value + 1 if key == 'Age' else value for key, value in my_dict.items()}
# Print the updated dictionary
print(new_dict)
{'Name': 'John', 'Age': 36, 'City': 'Calgary', 'Occupation': 'Engineer'}
new_dict = {key: value + 1 if key == 'Age' else value for key, value in my_dict.items()}
: In this line, a new dictionary named new_dict
is created using a dictionary comprehension. This comprehension iterates through the key-value pairs of the my_dict
dictionary using the items()
method. For each key-value pair, it checks if the key is “Age.” If the key is “Age,” it increments the value by 1; otherwise, it retains the original value. This results in a new dictionary with the same key-value pairs as my_dict
, except that the “Age” value has been increased by 1.
key: value + 1 if key == 'Age' else value
: This expression updates the value based on the condition. If the key is “Age,” it adds 1 to the value; otherwise, it keeps the original value.
3.3.2.6. Combining loops with conditionals#
# Iterate through the dictionary and check each key-value pair
# For example, let's print only the key-value pairs where the value is a string
for key, value in my_dict.items():
# Check if the value is a string using isinstance
if isinstance(value, str):
# If it's a string, print the key and value
print(key, ':', value)
Name : John
City : Calgary
Occupation : Engineer
These are some common techniques to loop through dictionaries in Python. Depending on your specific use case, you can choose the most appropriate method for your needs. Remember that dictionaries are unordered, so the order in which the elements are iterated may not match the order in which they were inserted.
3.3.3. Reverse lookup#
In Python, dictionaries support forward lookup (accessing the value using the key), but by design, they do not directly support reverse lookup (accessing the key using the value). However, you can achieve reverse lookup functionality using various approaches. Here are a couple of common methods to perform reverse lookup on a dictionary [Downey, 2015, Python Software Foundation, 2023]:
Example:
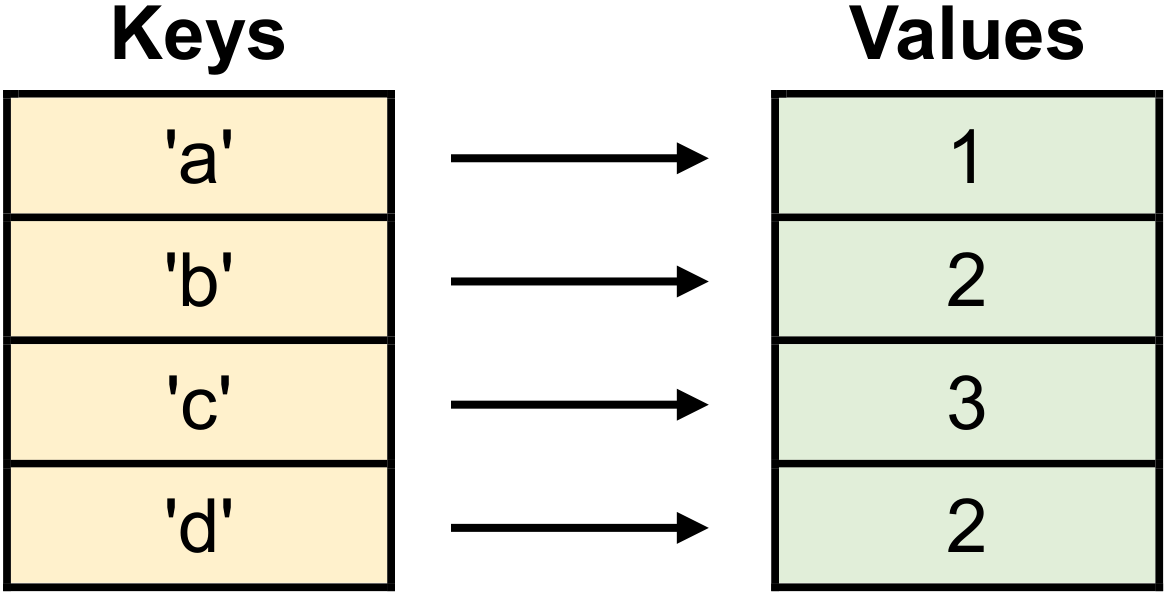
Fig. 3.8 A python dictionary.#
Method 1: Using a loop to find keys for a given value
# Define a function to perform reverse lookup in a dictionary
def reverse_lookup(d, value):
keys = [] # Initialize an empty list to store keys
for key, val in d.items(): # Iterate through key-value pairs in the dictionary
if val == value: # Check if the current value matches the target value
keys.append(key) # Append the current key to the list if it's a match
return keys # Return the list of keys
# Create a dictionary with key-value pairs
my_dict = {'a': 1, 'b': 2, 'c': 3, 'd': 2}
# Call the reverse_lookup function to find keys with a specific value
result = reverse_lookup(my_dict, 2)
# Print the result, which is a list of keys with the specified value
print(result) # Output: ['b', 'd']
['b', 'd']
Here’s how the code works:
def reverse_lookup(d, value):
: This line defines a function namedreverse_lookup
that takes two parameters:d
(a dictionary) andvalue
(the value to look up in the dictionary).keys = []
: This line initializes an empty list namedkeys
to store the keys that correspond to the givenvalue
.for key, val in d.items():
: This loop iterates through the items (key-value pairs) in the dictionaryd
.key
: During each iteration, this variable holds the current key from the dictionary.val
: During each iteration, this variable holds the corresponding value associated with the current key.
if val == value:
: Inside the loop, this condition checks if theval
(the value associated with the current key) is equal to thevalue
that you’re searching for.keys.append(key)
: If the condition is met (i.e., if the current value matches the targetvalue
), the current key is appended to thekeys
list.return keys
: After iterating through all the items in the dictionary, the function returns the list of keys that correspond to the specifiedvalue
.my_dict = {'a': 1, 'b': 2, 'c': 3, 'd': 2}
: This line initializes a dictionary namedmy_dict
with key-value pairs.result = reverse_lookup(my_dict, 2)
: Here, thereverse_lookup
function is called with the dictionarymy_dict
and the value2
as arguments. It will search for keys inmy_dict
that have the value2
and return them as a list.print(result)
: Finally, this line prints the result of thereverse_lookup
function, which contains the keys that have the value2
in the dictionary.# Output: ['b', 'd']
: This comment indicates the expected output of theprint
statement, which will display['b', 'd']
since both ‘b’ and ‘d’ in the dictionary have the value2
.
Method 2: Using a dictionary comprehension (Python 3.8+)
# Define a function to perform reverse lookup in a dictionary
def reverse_lookup(d, value):
keys = [] # Initialize an empty list to store keys
for key, val in d.items(): # Iterate through key-value pairs in the dictionary
if val == value: # Check if the current value matches the target value
keys.append(key) # Append the current key to the list if it's a match
return keys # Return the list of keys
# Create a dictionary with key-value pairs
my_dict = {'a': 1, 'b': 2, 'c': 3, 'd': 2}
# Call the reverse_lookup function to find keys with a specific value
result = reverse_lookup(my_dict, 2)
# Print the result, which is a list of keys with the specified value
print(result) # Output: ['b', 'd']
['b', 'd']
Here’s an explanation of each part of the code:
def reverse_lookup(d, value):
: This line defines a function namedreverse_lookup
that takes two parameters:d
(a dictionary) andvalue
(the value to look up in the dictionary).keys = []
: This line initializes an empty list namedkeys
to store the keys that correspond to the givenvalue
.for key, val in d.items():
: This loop iterates through the items (key-value pairs) in the dictionaryd
.key
: During each iteration, this variable holds the current key from the dictionary.val
: During each iteration, this variable holds the corresponding value associated with the current key.
if val == value:
: Inside the loop, this condition checks if theval
(the value associated with the current key) is equal to thevalue
that you’re searching for.keys.append(key)
: If the condition is met (i.e., if the current value matches the targetvalue
), the current key is appended to thekeys
list.return keys
: After iterating through all the items in the dictionary, the function returns the list of keys that correspond to the specifiedvalue
.my_dict = {'a': 1, 'b': 2, 'c': 3, 'd': 2}
: This line initializes a dictionary namedmy_dict
with key-value pairs.result = reverse_lookup(my_dict, 2)
: Here, thereverse_lookup
function is called with the dictionarymy_dict
and the value2
as arguments. It will search for keys inmy_dict
that have the value2
and return them as a list.print(result)
: Finally, this line prints the result of thereverse_lookup
function, which contains the keys that have the value2
in the dictionary.# Output: ['b', 'd']
: This comment indicates the expected output of theprint
statement, which will display['b', 'd']
since both ‘b’ and ‘d’ in the dictionary have the value2
.
Note
In both methods, the function reverse_lookup
takes a dictionary d
and a value as input and returns a list of keys that have the specified value. Note that these methods will return all the keys corresponding to the given value. If you expect only one key-value pair with the given value, you can modify the function to return just the first key found or handle multiple keys as needed.
Keep in mind that if you anticipate the need for reverse lookups frequently, you may want to consider maintaining a separate reverse dictionary that maps values to keys, especially if the dictionary is large, to optimize lookup performance.
3.3.4. Dictionaries and lists#
In Python, dictionaries and lists are two different built-in data structures that serve different purposes and have distinct characteristics. Let’s explore their differences and how they can be used [Downey, 2015, Python Software Foundation, 2023]:
3.3.4.1. Dictionaries:#
Dictionaries are unordered collections of key-value pairs.
They use a hash table to provide efficient lookup and insertion.
Keys must be unique and immutable (e.g., strings, numbers, or tuples), while values can be of any data type.
You can access, modify, add, and delete elements using their keys.
Dictionaries are typically used when you need to map keys to corresponding values and perform fast lookups based on those keys.
3.3.4.2. Lists:#
Lists are ordered collections of elements.
Elements in a list can be of any data type and can be duplicated.
You can access, modify, add, and delete elements in a list using their positions (indices).
Lists are useful when you need to store multiple items in a specific order, and you may perform operations like appending, slicing, or sorting.
3.3.4.3. Combining dictionaries and lists#
You can use dictionaries and lists together to represent more complex data structures.
For example, you can have lists of dictionaries or dictionaries containing lists.
Example: Example of a list of dictionaries:
people = [{'Name': 'John', 'Age': 30, 'Occupation': 'Engineer'},
{'Name': 'Jane', 'Age': 25, 'Occupation': 'Doctor'},
{'Name': 'Mike', 'Age': 35, 'Occupation': 'Teacher'}
]
Here’s an explanation of the code:
people
: This is a list that contains three dictionaries. Each dictionary represents a person’s information, and these dictionaries are enclosed in square brackets, indicating that they are elements of a list.Each dictionary within the list represents a person with three key-value pairs:
'Name'
: This key stores the person’s name as a string. For example, the first dictionary has'Name': 'John'
, indicating that the person’s name is John.'Age'
: This key stores the person’s age as an integer. For instance, the first dictionary has'Age': 30
, which means the person’s age is 30 years old.'Occupation'
: This key stores the person’s occupation as a string. For example, the first dictionary has'Occupation': 'Engineer'
, indicating that the person’s occupation is an engineer.
So, the people
list contains information about three individuals: John, Jane, and Mike, with their respective names, ages, and occupations stored in dictionaries within the list. This data structure is useful for organizing and storing information about multiple individuals or entities in a structured format.
Example: Example of a dictionary containing lists:
my_data = {'Numbers': [1, 2, 3, 4, 5],
'Fruits': ['Apple', 'Banana', 'Orange'],
'Colors': ['Red', 'Blue', 'Green']
}
Here’s an explanation of the code:
my_data
: This is a dictionary that contains three key-value pairs, enclosed within curly braces{}
.Each key in the dictionary represents a category, and each value is a list of items associated with that category. Here are the details of each key-value pair:
'Numbers'
: This key represents a category related to numbers. The associated value is a list[1, 2, 3, 4, 5]
, which contains five integers.'Fruits'
: This key represents a category related to fruits. The associated value is a list['Apple', 'Banana', 'Orange']
, which contains three strings, each representing a different fruit.'Colors'
: This key represents a category related to colors. The associated value is a list['Red', 'Blue', 'Green']
, which contains three strings, each representing a different color.
This data structure allows you to organize and store data in a hierarchical manner. It’s a convenient way to group related information, and you can access items within each category by using the corresponding key. For example, you can access the list of numbers as my_data['Numbers']
, which would return [1, 2, 3, 4, 5]
. Similarly, you can access the list of fruits as my_data['Fruits']
, and the list of colors as my_data['Colors']
.
3.3.4.4. Similarities and Differences Between Dictionaries and Lists#
Dictionaries and lists are two common data structures in programming, often used to store and manage collections of items. They have distinct characteristics that make them suitable for different purposes. Here are the similarities and differences between dictionaries and lists [Downey, 2015, Python Software Foundation, 2023]:
Similarities:
Collection of Items: Both dictionaries and lists are used to store multiple items or elements in a single data structure.
Mutable: Both dictionaries and lists are mutable, meaning you can modify their contents after creation. You can add, update, or remove items from them.
Iteration: You can iterate over the items in both dictionaries and lists using loops.
Mixed Data Types: Both data structures can store elements of various data types, such as strings, numbers, booleans, and even other data structures.
Differences:
Access by Key vs. Index: The primary difference between dictionaries and lists is how you access their elements. In a dictionary, elements are stored as key-value pairs, and you access an element using its associated key. In a list, elements are stored in a linear fashion, and you access an element using its index (position).
Ordering: Dictionaries in Python (prior to Python 3.7) were unordered collections, meaning the order of elements was not guaranteed. However, starting from Python 3.7, dictionaries maintain insertion order. Lists, on the other hand, maintain their order by default.
Duplication: In a dictionary, keys must be unique, while values can be duplicated. In a list, elements can be duplicated.
Usage: Dictionaries are often used when you have a set of unique keys that map to corresponding values, allowing for efficient lookups and retrieval of values based on keys. Lists are used when you need an ordered collection of items, and you primarily access them based on their position.
Size: Dictionaries are typically more memory-intensive than lists because they store both keys and values. Lists only store the values themselves.
Complexity: The time complexity for accessing an element in a dictionary is typically O(1) on average due to the hash-based indexing of keys. In a list, accessing an element by index has a time complexity of O(1), but searching for an element by value takes O(n) time on average, where n is the number of elements.
Example:
# Dictionary
student_info = {'Name': 'John',
'Age': 25,
'Grade': 'A'
}
# List
fruits = ['Apple', 'Banana', 'Orange']
# Accessing elements
student_name = student_info['Name']
second_fruit = fruits[1]
# Adding elements
student_info['City'] = 'Calgary'
fruits.append('Grape')
# Iterating through student_info dictionary:
for key, value in student_info.items():
print(key, '=',value)
Name = John
Age = 25
Grade = A
City = Calgary
# Iterating through fruits list:
for fruit in fruits:
print(fruit)
Apple
Banana
Orange
Grape