2.2. Iteration#
2.2.1. Reassignment#
In Python, reassignment refers to the process of changing the value of a variable after it has been assigned. Variables in Python are not fixed and can hold different values at different points in the program. You can reassign a variable multiple times within the same code block or even across different parts of your program [Python Software Foundation, 2023].
Here’s an example of reassigning a variable:
x = 10
print("x is initially:", x) # Output: x is initially: 10
x = 20
print("After reassignment, x is:", x) # Output: After reassignment, x is: 20
x = x + 5
print("After further reassignment, x is:", x) # Output: After further reassignment, x is: 25
x is initially: 10
After reassignment, x is: 20
After further reassignment, x is: 25
In this example, we first assign the value 10
to the variable x
. Then, we reassign x
to 20
, and finally, we reassign it again by adding 5
to its current value, resulting in 25
.
Reassignment is a powerful feature of Python that allows you to modify variables and update their values as your program runs. It is essential to keep track of the current value of a variable, especially when using it in calculations or conditional statements, as reassigning a variable will overwrite its previous value.
2.2.2. Updating variables#
In Python, you can update variables by performing operations on their current values. Updating variables is a form of reassignment, where you change the value of a variable based on its current value. It is a common practice when you want to modify the content of a variable during the course of your program [Downey, 2015, Python Software Foundation, 2023].
Here are some examples of updating variables using different operations:
2.2.2.1. Addition and Assignment#
x = 5
x += 3 # Update x by adding 3 to its current value
print(x) # Output: 8
8
2.2.2.2. Subtraction and Assignment#
y = 10
y -= 4 # Update y by subtracting 4 from its current value
print(y) # Output: 6
6
2.2.2.3. Multiplication and Assignment#
z = 2
z *= 5 # Update z by multiplying its current value by 5
print(z) # Output: 10
10
2.2.2.4. Division and Assignment#
a = 25
a /= 5 # Update a by dividing its current value by 5
print(a) # Output: 5.0 (result is a float due to division)
5.0
2.2.2.5. Modulus and Assignment#
b = 17
b %= 5 # Update b by taking the remainder of its current value divided by 5
print(b) # Output: 2
2
In each case, the variable is updated using a specific operation and its current value. These operations are commonly used when you want to increment or decrement the value of a variable or perform other calculations that involve the variable’s current state.
Command |
Description |
---|---|
|
Update |
|
Update |
|
Update |
|
Update |
|
Update |
Updating variables can be handy in various scenarios, such as counting occurrences, tracking values in loops, or storing intermediate results in complex calculations. Remember to consider the data type and the potential results when updating variables, as some operations may change the data type (e.g., division resulting in a float).
2.2.3. The while
statement#
In Python, the while
statement is used to create a loop that executes a block of code repeatedly as long as a certain condition remains true.
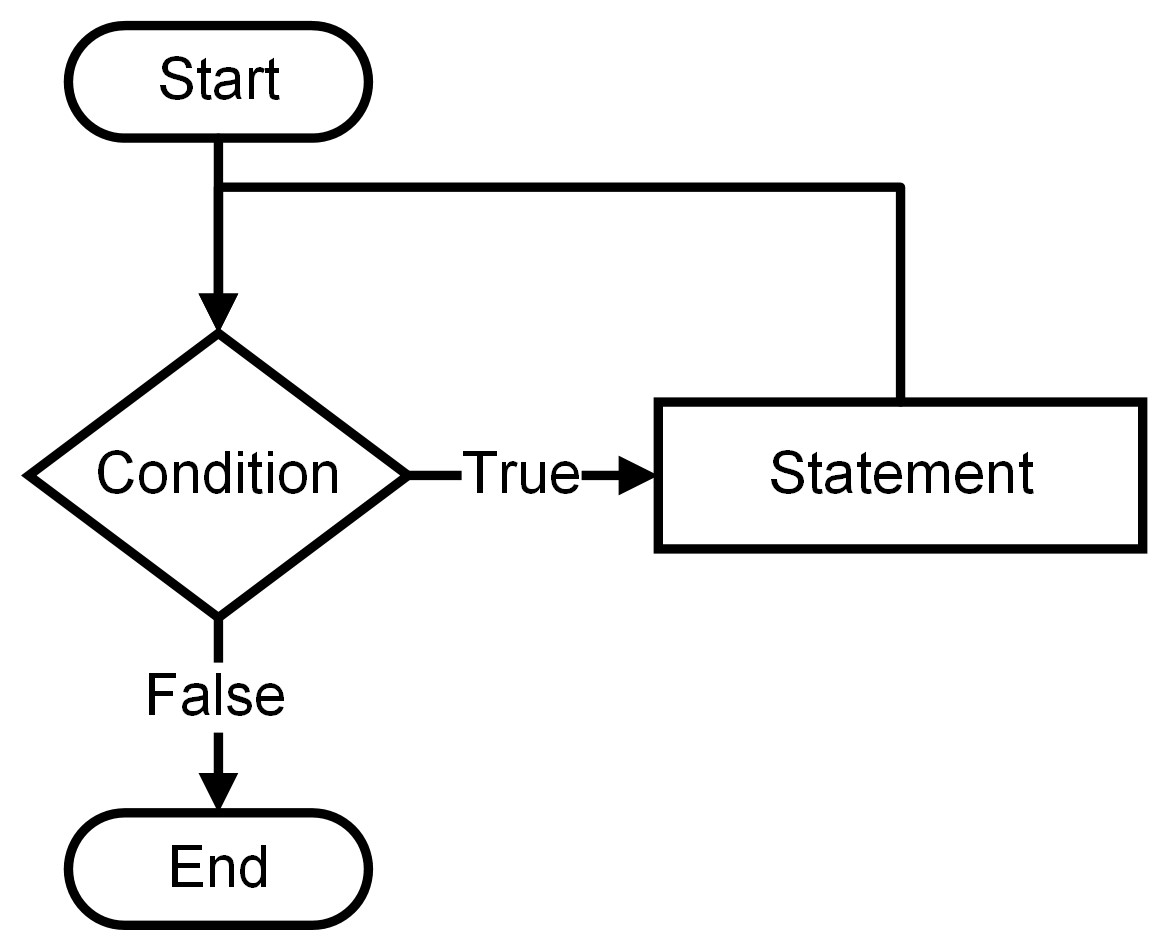
Fig. 2.2 Flowchart: The Structure of a ‘While’ Loop in Python.#
The general syntax of the while
loop is as follows:
while condition:
# Code block to be executed while the condition is True
# ...
The loop will keep executing the code block as long as the condition
remains true. Once the condition
becomes false, the loop will terminate, and the program will continue with the next line of code after the while
loop.
Here’s a simple example of using a while
loop to count from 1 to 5:
count = 1
while count <= 5:
print(count)
count += 1
1
2
3
4
5
In this example, the loop will keep executing the code block as long as the count
variable is less than or equal to 5. The variable count
starts with the value 1 and increments by 1 in each iteration until it reaches 6, at which point the condition count <= 5
becomes false, and the loop terminates.
It’s important to ensure that the condition in the while
loop eventually becomes false; otherwise, the loop will result in an infinite loop, causing the program to run indefinitely. You should include logic in the loop to modify the variables involved in the condition so that the condition becomes false at some point [Downey, 2015, Python Software Foundation, 2023].
Here’s an example of an infinite loop:
# Infinite loop (remove this code after running, as it will keep running indefinitely)
while True:
print("This is an infinite loop!")
In this example, the loop condition True
is always true, so the loop will never terminate, and “This is an infinite loop!” will be printed indefinitely. To stop the program from running, you can manually interrupt the execution (e.g., press Ctrl + C in the terminal or stop the program execution in the IDE).
2.2.4. break
#
In Python, the break
statement is used to terminate the execution of a loop prematurely. When the break
statement is encountered within a loop, the loop is immediately exited, and the program continues with the first statement after the loop [Downey, 2015, Python Software Foundation, 2023].
The break
statement is often used in combination with conditional statements (such as if
) to exit the loop based on a specific condition.
Here’s an example of using break
to stop a loop when a certain condition is met:
# Using break to stop the loop when the value becomes 5
count = 1
while count <= 10:
print(count)
if count == 5:
break
count += 1
1
2
3
4
5
In this example, the loop prints numbers from 1 to 10. However, when the value of count
becomes 5, the break
statement is executed, causing the loop to terminate prematurely. As a result, only numbers from 1 to 5 are printed.
The break
statement is particularly useful when you need to stop a loop based on a certain condition, rather than iterating through the entire range or collection. It helps improve the efficiency of your code by avoiding unnecessary iterations when the desired condition is met early in the loop.
2.2.5. The for
statement#
In Python, the for
statement is used to iterate over elements in a sequence (e.g., strings, lists, tuples, dictionaries) or any iterable object. The for
loop allows you to perform a specific block of code for each element in the sequence.
The general syntax of the for
loop is as follows [Downey, 2015, Python Software Foundation, 2023]:
for element in sequence:
# Code block to be executed for each element
# ...
Here’s an example of using the for
loop to iterate over a list:
numbers = [1, 2, 3, 4, 5]
for num in numbers:
print(num)
1
2
3
4
5
In this example, the for
loop iterates over each element in the list numbers
. For each iteration, the variable num
takes the value of the current element, and we print it on a separate line.
You can also use the for
loop to iterate over a string:
text = "Hello, Calgary!"
for char in text:
print(char)
H
e
l
l
o
,
C
a
l
g
a
r
y
!
The for
loop is not limited to lists and strings; you can use it with any iterable object, including tuples, dictionaries, and even custom objects that support iteration.
In the case of dictionaries, you can use the for
loop to iterate over keys, values, or items (key-value pairs):
student_scores = {"Alice": 85, "Bob": 92, "Charlie": 78}
for name,score in student_scores.items():
print(f"{name}: {score}")
Alice: 85
Bob: 92
Charlie: 78
The for
loop is a powerful and versatile tool in Python for processing sequences and collections of data. It simplifies the process of iterating over elements and is commonly used in various programming tasks.
Summary:
Loop Type |
Description |
---|---|
|
Executes a block of code a specified number of times, iterating over a sequence (such as a list, tuple, or range) or any iterable object. |
|
Repeatedly executes a block of code as long as a given condition is true. The loop continues until the condition becomes false. |
Note
Discussion on dictionaries will be covered in Chapter 3.