7.1. Getting started with Matplotlib#
Matplotlib is a powerful Python library used to create a wide range of visualizations and plots. It has garnered widespread popularity for its role in facilitating data visualization and exploration tasks. To commence your exploration of Matplotlib’s functionalities, adhere to these essential guidelines [Pajankar, 2021, Matplotlib Developers, 2023]:
7.1.1. Install Matplotlib#
If you don’t have Matplotlib installed already, you can install it using pip
by running the following command in your terminal or command prompt:
>>> pip install matplotlib
Remark
Remember that Google Colab includes several well-known data science libraries already installed, such as NumPy, Pandas, Matplotlib, and others.
7.1.2. Import Matplotlib#
In your Python script or Jupyter Notebook, start by importing Matplotlib:
import matplotlib.pyplot as plt
7.1.3. Create Basic Plots#
Here are examples of some basic types of plots you can create using Matplotlib:
7.1.3.1. Line Plot#
A line plot is useful for visualizing data points connected by lines, typically used for showing trends over time or continuous data. You can access the full description of the function here.
Example - Simple:
import matplotlib.pyplot as plt
# Sample data
x = [1, 2, 3, 4, 5]
y = [2, 4, 6, 8, 10]
# Create a line plot
plt.plot(x, y, marker='o')
# Add labels and title
plt.xlabel('X-axis')
plt.ylabel('Y-axis')
plt.title('Line Plot Example')
# Display the plot
plt.show()
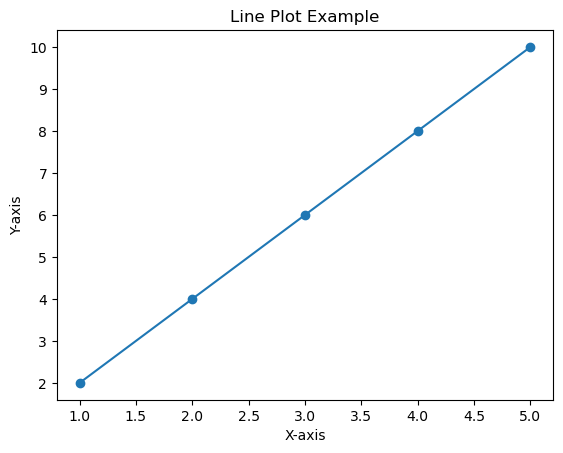
Example - Advanced:
import matplotlib.pyplot as plt
# Define data
x, y = [1, 2, 3, 4, 5], [2, 4, 6, 8, 10]
# Create a figure and axis with custom style
fig, ax = plt.subplots(figsize=(6, 4.5))
# Create a line plot with custom markers and colors
line, = ax.plot(x, y, 'o-', color='#FF5733', linewidth=4,
markersize=8, label='Data Points',
markerfacecolor='#e8eafc',
markeredgecolor = '#2236e1',
markeredgewidth=3)
# Annotate each data point with its value
for i, j in zip(x, y):
ax.annotate(f'({i},{j})', (i, j), textcoords="offset points", xytext=(-10, 10), ha='center', fontsize=10, color='black')
# Set various customization settings using ax.set()
ax.set(
xlabel='X-axis', ylabel='Y-axis', title='Fancy Line Plot Example', facecolor='#F2F4F7',
xticks=x, yticks=y,
xlim=(min(x) - 0.5, max(x) + 0.5),
ylim=(min(y) - 1, max(y) + 1),
xscale='linear', yscale='linear', frame_on=True
)
# Remove top and right spines, customize tick parameters
ax.spines['top'].set_visible(False)
ax.spines['right'].set_visible(False)
ax.tick_params(axis='both', which='both', direction='out', colors='#65737E', labelsize=10)
# Customize grid
ax.grid(True, linestyle='--', linewidth=0.5, alpha=0.7, color='#65737E')
# Add legend with custom style
ax.legend(handles=[line], loc='upper left', fontsize=12)
# Adjust layout and display the plot
plt.tight_layout()
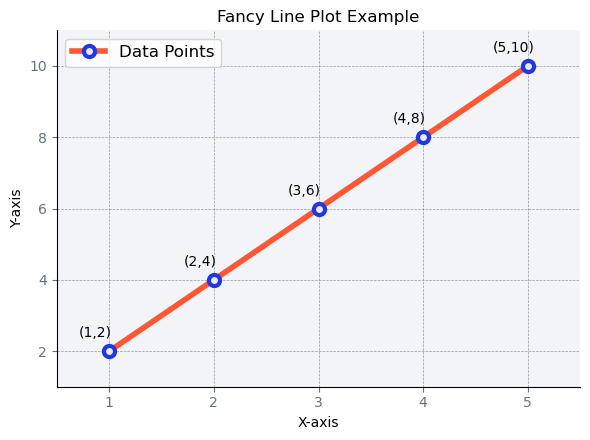
Note
This serves as a mere illustration of a line plot generated using matplotlib. Throughout this chapter, we will delve into the necessary stages to create more intricate plots.
7.1.3.2. Scatter Plot#
A scatter plot is used to display individual data points as markers without connecting lines, great for visualizing relationships between two variables. You can access the full description of the function here.
Example - Simple:
import matplotlib.pyplot as plt
# Sample data
x = [1, 2, 3, 4, 5]
y = [2, 4, 6, 8, 10]
# Create a scatter plot
plt.scatter(x, y, marker='o', color='blue')
# Add labels and title
plt.xlabel('X-axis')
plt.ylabel('Y-axis')
plt.title('Scatter Plot Example')
# Display the plot
plt.show()
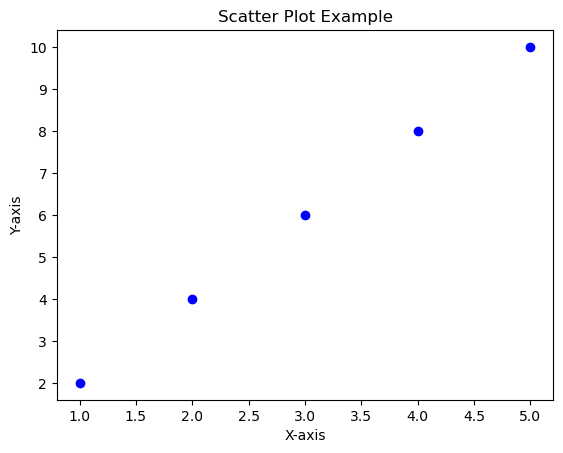
Example - Advanced:
import matplotlib.pyplot as plt
# Sample data
x = [1, 2, 3, 4, 5]
y = [2, 4, 6, 8, 10]
# Create a figure and axis with custom style
fig, ax = plt.subplots(figsize=(6, 4.5))
# Create a scatter plot with custom markers and colors
scatter = ax.scatter(x, y, marker='o', fc='#3498DB', ec = 'Navy', lw =1, s=100, label='Data Points')
# Annotate each data point with its value
for i, j in zip(x, y):
ax.annotate(f'({i},{j})', (i, j), textcoords="offset points", xytext=(-10,10), ha='center', fontsize=10, color='black')
# Set various customization settings using ax.set()
ax.set(
xlabel='X-axis', ylabel='Y-axis', title='Fancy Scatter Plot Example',
facecolor='#F2F4F7', # Background color
xlim=(min(x) - 0.5, max(x) + 0.5), # X-axis limits
ylim=(min(y) - 1, max(y) + 1), # Y-axis limits
xscale='linear', yscale='linear', # Axis scales
frame_on=True, # Show plot frame
xticks=x, yticks=y, # Ticks
)
# Remove top and right spines, customize tick parameters
ax.spines['top'].set_visible(False)
ax.spines['right'].set_visible(False)
ax.tick_params(axis='both', which='both', direction='out', colors='#65737E', labelsize=10)
# Customize grid
ax.grid(True, linestyle='--', linewidth=0.5, alpha=0.7, color='#65737E')
# Add legend with custom style
ax.legend(handles=[scatter], loc='upper left', fontsize=12)
# Display the plot
plt.tight_layout()
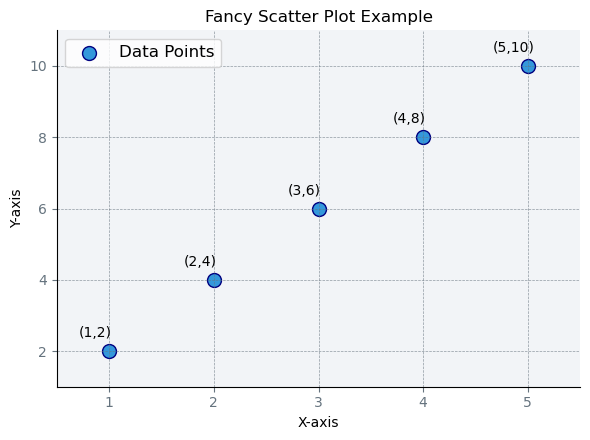
7.1.3.3. Bar Plot#
A bar plot is useful for comparing data among different categories or groups. You can access the full description of the function here.
Example - Simple:
import matplotlib.pyplot as plt
# Sample data
categories = ['Category A', 'Category B', 'Category C']
values = [10, 15, 7]
# Create a bar plot
plt.bar(categories, values, color='green')
# Add labels and title
plt.xlabel('Categories')
plt.ylabel('Values')
plt.title('Bar Plot Example')
# Display the plot
plt.show()
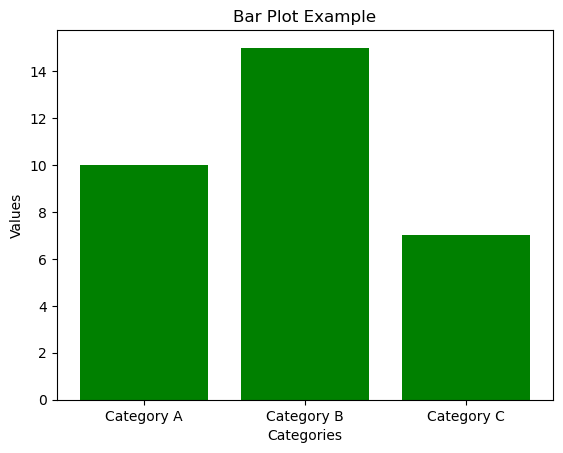
Example - Advanced:
import matplotlib.pyplot as plt
# Sample data
categories = ['Category A', 'Category B', 'Category C']
values = [10, 15, 7]
# Create a figure and axis with custom style
fig, ax = plt.subplots(figsize=(4, 4.5))
# Create a bar plot with custom colors
bars = ax.bar(categories, values, fc='YellowGreen', ec = 'DarkGreen', lw = 2, hatch = '\\')
# Annotate each bar with its value
for bar in bars:
height = bar.get_height()
ax.annotate(f'{height}', xy=(bar.get_x() + bar.get_width() / 2, height),
xytext=(0, 3), textcoords="offset points", ha='center', fontsize=10, color='black')
# Set various customization settings using ax.set()
ax.set(
xlabel='Categories', ylabel='Values', title='Fancy Bar Plot Example',
facecolor='#F2F4F7', # Background color
xticks=[], yticks=values, # Ticks
xlim=(-0.5, len(categories) - 0.5), # X-axis limits
ylim=(0, max(values) + 1), # Y-axis limits
xscale='linear', yscale='linear', # Axis scales
frame_on=True, # Show plot frame
)
# Remove top and right spines, customize tick parameters
ax.spines['top'].set_visible(False)
ax.spines['right'].set_visible(False)
ax.tick_params(axis='both', which='both', direction='out', colors='#65737E', labelsize=10)
# Customize grid
ax.grid(True, linestyle='--', linewidth=0.5, alpha=0.7, color='#65737E')
# Display the plot
plt.tight_layout()
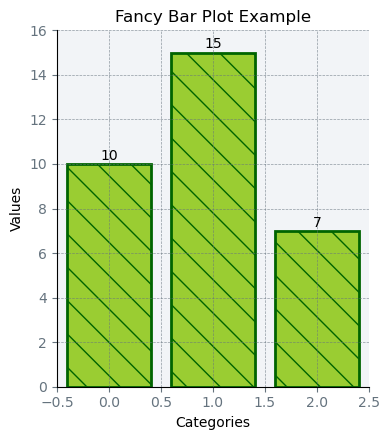
These are just a few examples of basic plots you can create using Matplotlib. The library provides extensive customization options for labels, titles, colors, and more. As you become more comfortable with Matplotlib, you can explore other types of plots, such as histograms, pie charts, and more advanced visualizations.
Note
The plots presented in this section serve as exemplars for their respective plot categories. By the end of this chapter, you will have gained the skills required to create more complex and aesthetically refined plots. These visualizations are provided as didactic tools to illustrate foundational methodologies.